Since the release of iOS 10.3 developers are able to change the app icon from your app programatically. Although it can’t be used to create a dynamic icon (like the Calendar or Clock-app), we are given the opportunity to ship the app with more than one kind of icon so the user will be able to change that. The official MLB At Bat and NHL apps on iOS used this to change the icon of their app to change the app’s icon to their favorite team’s logo.
Xamarin released their support of iOS 10.3 pretty quickly after the release from Apple, making this functionality available for Xamarin.iOS developers as well (they called it adaptive icons). Let’s see how we can use this to set alternate app icons in iOS using Xamarin.
The goal in the sample would be that we’ll be able to change the app icon of the app so the user can choose between three different icons to be displayed on the springboard. We’ll be using the setAlternateIconName
method that’s available in iOS 10.3 to achieve this goal. The source code can be found on Github in case you directly want to dive into the code. Otherwise, read on!
What we’ll be creating
Keeping the requirements stated above in mind, this is what we’ll be creating:
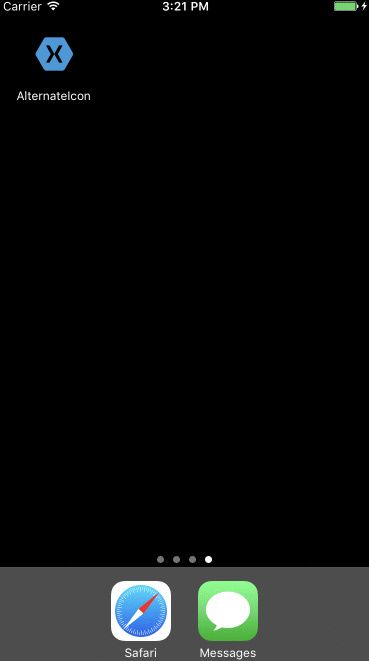
This turns out to be pretty easy! Let’s take a look on how you’ll be able to create this as well.
Prerequisites
- Xcode 8.3 which will bring iOS 10.3 support.
- Visual Studio or Xamarin Studio with Xcode 8.3 support.
- The icon files for all the app icons in 60pt@2x and 60pt@3x (when doing iPhone support only).
These are the icons that I’ll be using in the demo:
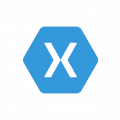


I’ll be using Xamarin Studio in this sample and created a simple 3-button layout to change the app icons. Check out the source on Github to check how the layout looks and is bound to the code. Now that we have everything in place, let’s see how we can change our app icon!
Resources
First, let’s add the default app icon. Open up the Assets.xcassets
file and add the iPhone App (2x & 3x) icons that you’ll be using as the default.

I’ll be using the Xamarin-icon as default that’ll later change to the Microsoft or Marcofolio icons. So far, we haven’t done anything new – this is just the way icons are set for iOS.
Add the alternate icons to the Resources
-folder of the project. To make use of the them, we’ll need to open up the Info.plist
and add the CFBundleAlternateIcons
to the CFBundleIcons
-section of the file like so:
<key>CFBundleIcons</key>
<dict>
<key>CFBundleAlternateIcons</key>
<dict>
<key>Microsoft</key>
<dict>
<key>CFBundleIconFiles</key>
<array>
<string>Microsoft</string>
</array>
<key>UIPrerenderedIcon</key>
<false/>
</dict>
<!-- More alternate icons -->
</dict>
</dict>
When viewing your Info.plist
-file in Source-mode, you should see something like this:
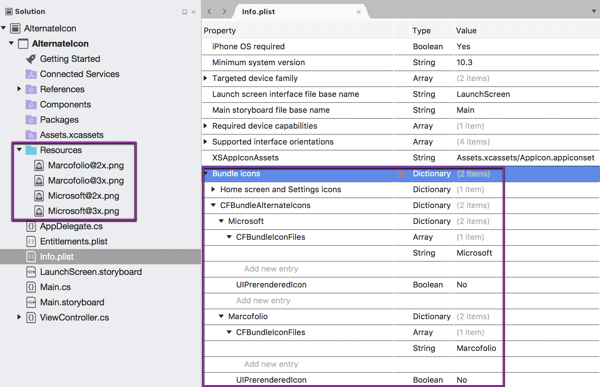
Take note of the Key
and item in the Array
(in my example both Microsoft
). Those are the names of the icons and will be used in the code as well.
Code
Now that we have everything in place, we can move forward to actually change the icon. This is actually a pretty simple part, since we can use the supportsAlternateIcons
& setAlternateIconName
functionality to get what we want.
First, we’ll have to check if the OS does actually support the use of alternate icons by checking the SupportsAlternateIcons
property. For demo purposes we throw an Exception
if it doesn’t, but you’d want to fall back to your default icon when using this in an actual app.
private void SupportsAlternateIcons(Action callback) {
if (UIApplication.SharedApplication.SupportsAlternateIcons) {
callback();
}
else {
throw new Exception("iOS version doesn't support AlternateIcons.");
}
}
And the last part is to utilize the SetAlternateIconName
-method to change the icon. We’ll add some basic error handling for demo purposes, but I hope you get the idea.
private static string AlternateIconNameMicrosoft = "Microsoft";
partial void BtnIconXamarin_TouchUpInside(UIButton sender) {
SupportsAlternateIcons(() =>
{
UIApplication.SharedApplication.SetAlternateIconName(null, HandleError); // Default icon
});
}
partial void BtnIconMicrosoft_TouchUpInside(UIButton sender) {
SupportsAlternateIcons(() =>
{
UIApplication.SharedApplication.SetAlternateIconName(AlternateIconNameMicrosoft, HandleError);
});
}
private void HandleError(Foundation.NSError error) {
if (error != null) {
throw new Exception($"Can't change Icon. Error: {error.Description}.");
}
}
When passing null
as the first parameter, the icon changes back to the default. When passing the icon name (as a string
), make sure it’s the same as the key
in your Info.plist
file.
Conclusion
Run the project and check if you’re able to change your app icon. The user should get a message the icon will change, but after accepting the new icon sits right where you expect it. It’s just that simple!
Don’t forget to poke around in the example code on Github and tell me what you think through Twitter!
2 comments On Set alternate app icons in iOS using Xamarin
Can do the same for Android ?
Sadly, no since it’s not an official Android feature. There are some hacky workarounds, but they tend to be buggy and work differently across different versions of Android.